How to Manipulate Colors in JavaScript Using Chroma.js

In today’s tutorial screencast I’m going to show you how to manipulate colors with JavaScript. That’s right, JavaScript. Not CSS. Let’s dive in to see what I’m talking about!
Watch Screencast
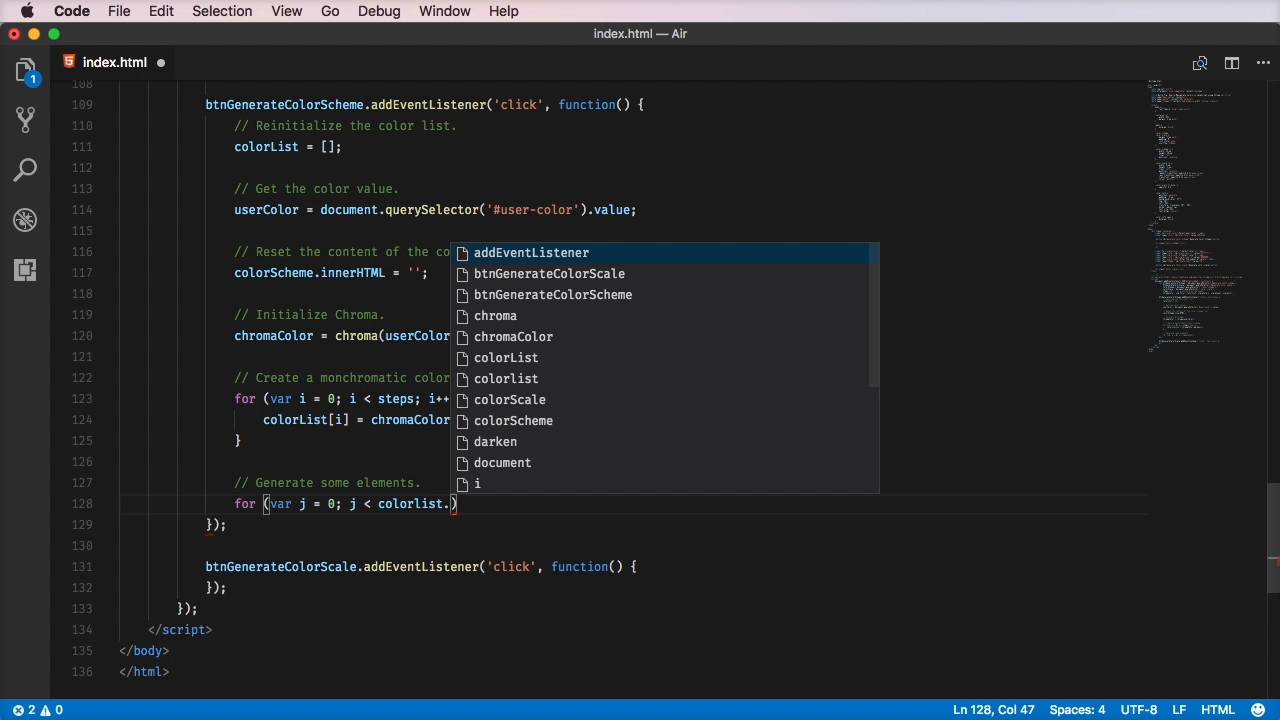
We’ve all become used to the idea that CSS handles styles whilst JavaScript is used for behavior, and the two concerns should be kept separate. But when it’s not possible to define styles directly via CSS, such as when they’re set via user input, JavaScript will do all the heavy lifting for you.
Chroma.js is a small library which can be a big help with manipulating colors, so let’s see how to get started using it.
Hook it Up
Chroma can be pulled from its repo on Github, or linked to from CDNJS using this link:
1 |
<script src="https://cdnjs.cloudflare.com/ajax/libs/chroma-js/1.3.4/chroma.min.js"></script> |
Demo
Take a look at the quick demo I built to show you what’s possible. We’re going to build a color scheme generator which takes an inputted color value and uses it to output a monochromatic scheme.
If you want to follow along while I recreate the demo, grab the start.html file (which contains everything but the JavaScript we’re going to write) and open it up in a code editor.



Begin by adding the following chunk of code between the <script>
tags at the bottom of the html document:
1 |
document.addEventListener('DOMContentLoaded', function() { |
2 |
|
3 |
var btnGenerateColorScheme = document.querySelector('#generate-color-scheme'), |
4 |
btnGenerateColorScale = document.querySelector('#generate-color-scale'), |
5 |
colorScheme = document.querySelector('.color-scheme'), |
6 |
colorScale = document.querySelector('.color-scale'), |
7 |
steps = 5, // is preferably an odd number |
8 |
chromaColor, userColor, colorList, scaleColors, scaleCount, scaleList; |
9 |
|
10 |
btnGenerateColorScheme.addEventListener('click', function() { |
11 |
|
12 |
});
|
13 |
|
14 |
btnGenerateColorScale.addEventListener('click', function() { |
15 |
|
16 |
});
|
17 |
|
18 |
});
|
This is a big chunk, but is much less intimidating than you may at first think! It begins with an event listener DOMContentLoaded
which waits until the document is loaded before executing any of the function which follows.
Next I define a bunch of variables which we’re going to use, starting with two buttons, then the colorScheme
and colorScale
containers, then steps
(a number we’ll use shortly).
Lastly, there are two click event listeners with (as yet) empty functions.We’re going to begin with the first of these, the btnGenerateColorScheme
, which is (unsurprisingly) the button which will generate a color scheme.
btnGenerateColorScheme
Within the empty function, between the curly braces, we’ll start by setting an empty array called colorList
:
1 |
// Reinitialize the color list.
|
2 |
colorList = []; |
When a user clicks the button the first thing we need to do is get the color from the #user-color
input.
1 |
// Get the color value.
|
2 |
userColor = document.querySelector('#user-color').value; |
Then we need to reset the values within the color scheme list. This is done by stripping the innerHTML
of the .color-scheme
element, which is in our case an <ol>
. Here you can see we’re making the innerHTML equal an empty string.
1 |
// Reset the content of the color scheme list.
|
2 |
colorScheme.innerHTML = ''; |
Calling Chroma
Now for the fun part! We initialize the chroma.js library by calling chroma()
and passing in a color:
1 |
// Initialize Chroma.
|
2 |
chromaColor = chroma(userColor); |
Our color is userColor
, the value we pulled from the input earlier.
We’re going to create a monochromatic color scheme based on the chosen color. We’ll do this by creating an array, thanks to the following for
loop:
1 |
// Create a monchromatic color scheme.
|
2 |
for (var i = 0; i < steps; i++) { |
3 |
colorList[i] = chromaColor.darken(i); |
4 |
}
|
This loop iterates over the number of steps defined in the variable steps
(we set it to 5 earlier on, remember?) Each time we iterate, a slightly darker shade is added to the collection.
Finally, we need to generate some elements using the five values in our array. Each time we create a <li>
element, give it a backgroundColor
according to our colorList
, then append it to colorScheme
.
1 |
// Generate some elements.
|
2 |
for (var j = 0; j < colorList.length; j++) { |
3 |
var newItem = document.createElement('li'); |
4 |
|
5 |
newItem.style.backgroundColor = colorList[j]; |
6 |
|
7 |
colorScheme.appendChild(newItem); |
8 |
}
|
Done!
We’ve successfully built a color scheme generator which outputs a series of blocks, starting with the picked color and gradually getting darker.



You might want to improve this by having the picked color placed in the middle of the range with lighter colors to the left and darker colors to the right, in which case take a look at the video and follow along with the final steps. You could also add color details for each block, such as the hex value; I also explain this in the screencast.
Lastly, the screencast also includes instructions for building the color scale generator, so jump in and let me know how you get on!
Further Resources
- chroma.js on Github
- Its creator @driven_by_data (Gregor Aisch) on Twitter
- Starter file for this tutorial